LangGraph Agent with MCP adapter
This example demonstrates how to build a LangGraph agent that connects to a local MCP server, then wraps the agent as a uAgent and registers it on Agentverse using uAgents-adapter for discovery and use by ASI:One LLM.
Overview
- LangGraph agent connects to a local MCP server using
langchain_mcp_adapters
. - The agent is wrapped using
uagents_adapter
to become a uAgent. - The uAgent is registered on Agentverse, making it discoverable and callable by ASI:One LLM.
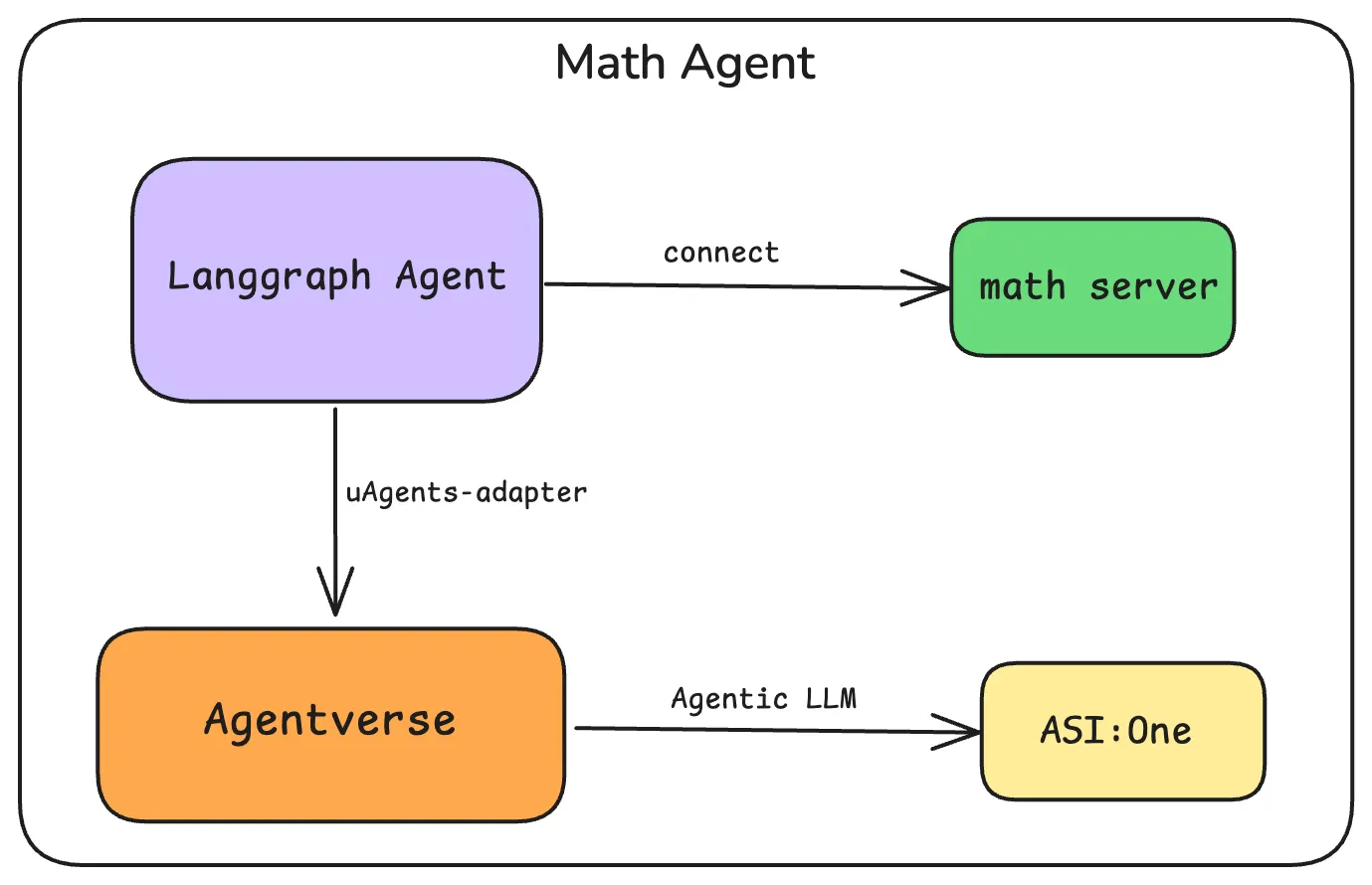
Example: Math MCP Server Integration
1. Create the Math MCP Server
math_server.py
from mcp.server.fastmcp import FastMCP
mcp = FastMCP("Math")
@mcp.tool()
def add(a: int, b: int) -> int:
"""Add two numbers"""
return a + b
@mcp.tool()
def multiply(a: int, b: int) -> int:
"""Multiply two numbers"""
return a * b
if __name__ == "__main__":
mcp.run(transport="stdio")
2. Create and Register the LangGraph Agent
agent.py
import os
import time
from dotenv import load_dotenv
from langchain_openai import ChatOpenAI
from langchain_core.messages import HumanMessage
from mcp import ClientSession, StdioServerParameters
from mcp.client.stdio import stdio_client
import asyncio
from langchain_mcp_adapters.tools import load_mcp_tools
from langgraph.prebuilt import create_react_agent
from uagents_adapter import LangchainRegisterTool, cleanup_uagent
from uagents_adapter.langchain import AgentManager
# Load environment variables
load_dotenv()
# Set your API keys
OPENAI_API_KEY = os.getenv("OPENAI_API_KEY")
API_TOKEN = os.getenv("AGENTVERSE_API_KEY")
# Initialize the model
model = ChatOpenAI(model="gpt-4o")
# Store the agent globally so it can be accessed by the wrapper function
agent = None
async def setup_math_agent():
global agent
print("Setting up math agent...")
server_params = StdioServerParameters(
command="python",
args=["math_server.py"],
)
async with stdio_client(server_params) as (read, write):
async with ClientSession(read, write) as session:
await session.initialize()
tools = await load_mcp_tools(session)
agent = create_react_agent(model, tools)
# Test the agent
test_response = await agent.ainvoke({
"messages": [HumanMessage(content="what's (3 + 5) x 12?")]
})
print(f"Test response: {test_response['messages'][-1].content}")
# Keep the connection alive
while True:
await asyncio.sleep(1)
def main():
# Initialize agent manager
manager = AgentManager()
# Create agent wrapper
async def agent_func(x):
response = await agent.ainvoke({"messages": [HumanMessage(content=x)]})
return response["messages"][-1].content
agent_wrapper = manager.create_agent_wrapper(agent_func)
# Start the agent in background
manager.start_agent(setup_math_agent)
# Register with uAgents
print("Registering math agent...")
tool = LangchainRegisterTool()
agent_info = tool.invoke(
{
"agent_obj": agent_wrapper,
"name": "math_agent_langchain_mcp",
"port": 8080,
"description": "A math calculation agent",
"api_token": API_TOKEN,
"mailbox": True
}
)
print(f"✅ Registered math agent: {agent_info}")
try:
manager.run_forever()
except KeyboardInterrupt:
print("🛑 Shutting down...")
cleanup_uagent("math_agent")
print("✅ Agent stopped.")
if __name__ == "__main__":
main()
Getting Started
-
Set up environment variables in a
.env
file:OPENAI_API_KEY=your_openai_api_key
AGENTVERSE_API_KEY=your_agentverse_api_key -
Install dependencies:
pip install langchain-openai langgraph mcp langchain-mcp-adapters uagents-adapter python-dotenv
-
Create the files:
- Save the math server code as
math_server.py
- Save the agent code as
agent.py
- Save the math server code as
-
Run the agent:
python agent.py
-
Test your agent by querying it from ASI:One LLM or the chat Agentverse UI.
- Open your Agentverse account and goto local agents.
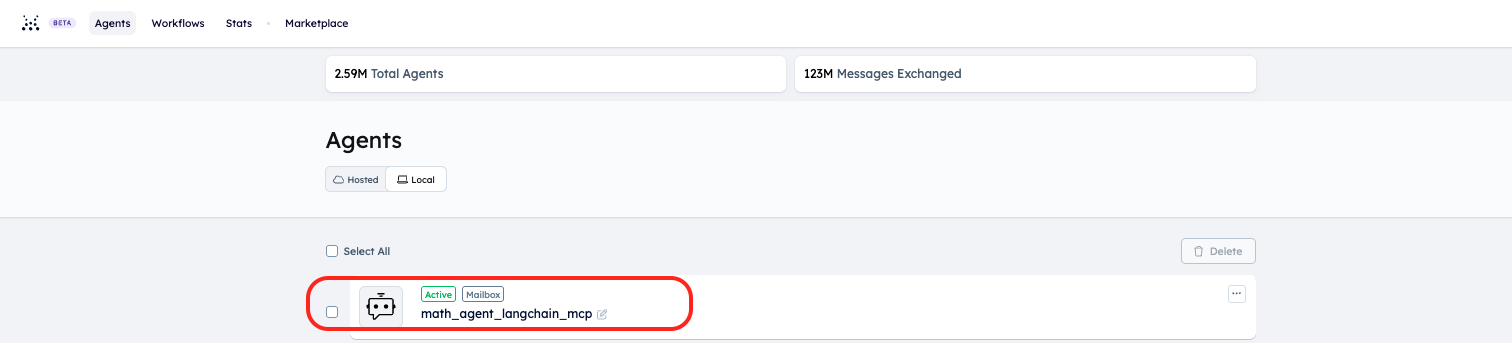
- Click on your agent and Use the "Chat with Agent" button on your Agentverse agent page
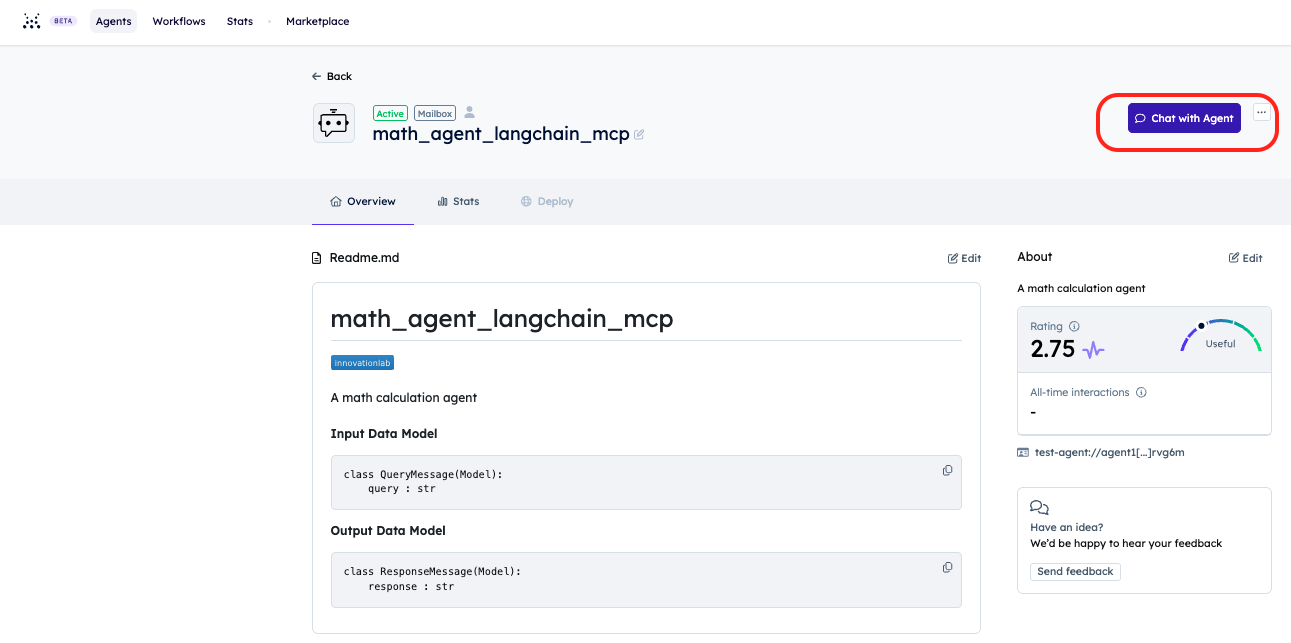

- Agent logs:
(venv) xyz@Fetchs-MacBook-Pro mcp-langgraph-test % python3 agent.py
Initializing agent...
Setting up math agent...
Processing request of type ListToolsRequest
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
Processing request of type CallToolRequest
Processing request of type CallToolRequest
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
Test response: \((3 + 5) \times 12 = 8 \times 12 = 96\).
Registering math agent...
INFO: [math_agent_langchain_mcp]: Starting agent with address: agent1qdspvt6tm34n3dnau9yekp8f3axmhd0vsmacxy59q20enmtvfvn0uxrvg6m
INFO: [math_agent_langchain_mcp]: Agent 'math_agent_langchain_mcp' started with address: agent1qdspvt6tm34n3dnau9yekp8f3axmhd0vsmacxy59q20enmtvfvn0uxrvg6m
INFO: [math_agent_langchain_mcp]: Agent inspector available at https://agentverse.ai/inspect/?uri=http%3A//127.0.0.1%3A8080&address=agent1qdspvt6tm34n3dnau9yekp8f3axmhd0vsmacxy59q20enmtvfvn0uxrvg6m
INFO: [math_agent_langchain_mcp]: Starting server on http://0.0.0.0:8080 (Press CTRL+C to quit)
INFO: [math_agent_langchain_mcp]: Starting mailbox client for https://agentverse.ai
INFO: [math_agent_langchain_mcp]: Mailbox access token acquired
INFO: [uagents.registration]: Registration on Almanac API successful
WARNING: [uagents.registration]: Mismatch in almanac contract versions: supported (2.1.0), deployed (2.3.0). Update uAgents to the latest version for compatibility.
INFO: [uagents.registration]: Almanac contract registration is up to date!
✅ Registered math agent: Created uAgent 'math_agent_langchain_mcp' with address agent1qdspvt6tm34n3dnau9yekp8f3axmhd0vsmacxy59q20enmtvfvn0uxrvg6m on port 8080
Connecting agent 'math_agent_langchain_mcp' to Agentverse...
INFO: [mailbox]: Successfully registered as mailbox agent in Agentverse
Successfully connected agent 'math_agent_langchain_mcp' to Agentverse
Updating agent 'math_agent_langchain_mcp' README on Agentverse...
Successfully updated agent 'math_agent_langchain_mcp' README on Agentverse
INFO: [math_agent_langchain_mcp]: Got a message from agent1qwy49pqq7zpg0d2ygv72wdr05vf4kuzc80lmnr9zev6h7vkdq3dk74rdxuq
INFO: [math_agent_langchain_mcp]: Got a text message from agent1qwy49pqq7zpg0d2ygv72wdr05vf4kuzc80lmnr9zev6h7vkdq3dk74rdxuq: can you do calculation 7 + 9 - 8 * 6 + 89 - 6 + 9 * 10 ?
INFO: [math_agent_langchain_mcp]: Sending structured output prompt to {'title': 'QueryMessage', 'type': 'object', 'properties': {'query': {'title': 'Query', 'type': 'string'}}, 'required': ['query']}
INFO: [math_agent_langchain_mcp]: Sent structured output prompt to agent1q0h70caed8ax769shpemapzkyk65uscw4xwk6dc4t3emvp5jdcvqs9xs32y
INFO: [math_agent_langchain_mcp]: Received structured output response from agent1q0h70caed8ax769shpemapzkyk65uscw4xwk6dc4t3emvp5jdcvqs9xs32y: 7 + 9 - 8 * 6 + 89 - 6 + 9 * 10 = ?
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
Processing request of type CallToolRequest
Processing request of type CallToolRequest
Processing request of type CallToolRequest
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
Processing request of type CallToolRequest
Processing request of type CallToolRequest
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
Processing request of type CallToolRequest
INFO:httpx:HTTP Request: POST https://api.openai.com/v1/chat/completions "HTTP/1.1 200 OK"
note
Note: This example demonstrates a simple math agent, but you can extend it to use multiple MCP servers. For more advanced examples, check out:
- Multi-Server Agent - Shows how to connect to multiple MCP servers (math and weather) using
MultiServerMCPClient
.